Python3 hackerRank exercise with solutions (Set 3)
1. Computing paradox :
You are provided with a playlist of N songs, each has a unique positive integer length. Assume that you like all songs in the playlist, but there would be a song that you like more than other songs. It is named as computing paradox.
You decided to sort the songs in increasing order of song length. For example , if the length of the songs in a list are {1,3,5,2,4} after sorting it becomes {1,2,3,4,5}. Before sorting , "Computing paradox" was on k_th position in the playlist.
Your task is to find the position of "Computing paradox" in the sorted playlist.
Input format
The first line contains the number N denoting the number of songs in the playlist.
The second line contains N space separated integers A1,A2,....An denoting the length of each song.
The third line contains an integer k , denoting the position of the Computing paradox in the initial playlist.
Output format
A single line containing the position of Computing paradox in the sorted playlist.
Example :
Input
4
1 3 4 2
2
Output
3
Explanation
N equals to 4 , k equals to 2 , A={1,3,4,2} , the answer is 3 because the element 4 in A is present in 3rd index on the sorted list of A
Code
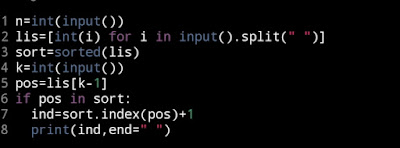
Output
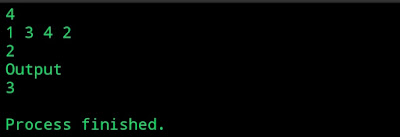
2. Swap the case :
Given a string you have to convert all lowercase letters to upper case and vice versa.
Input format
A single line containing the string S.
Output format
Print the modified string
Sample input
Hello world!
Sample Output
hELLO wORLD!
Code

Output
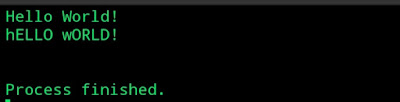
3. First and last :
Write a python program to print a string made of first 2 and last 2 characters of the given string. If the string length is less than 2 , return instead an empty string.
Input format
The first line of the input contains the string
S.
Output format
The first line of the output contains the modified string.
Sample input
Programming
Sample Output
Prng
Code
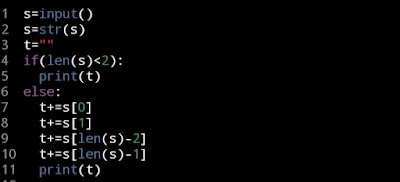
Output
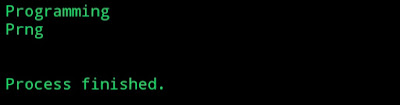
4. Rotate the matrix :
Given a square matrix of order n, you have to write a python program to rotate this matrix such that each element is shifted by one place in a clockwise manner.
Input format
The first line of the input contains a number representing the number of rows and columns.
After this, there are n rows with each row containing n elements separated by space.
Output format
Print the elements of the modified matrix with each row in a new line and all the elements in each row separated by space.
Sample input
3
1 2 3
4 5 6
7 8 9
Sample Output
4 1 2
7 5 3
8 9 6
Explanation
In the above example, there is an odd number of rows and columns. Hence excluding the middle element i.e. 5 all elements were shifted to one position in a clockwise manner.
Code
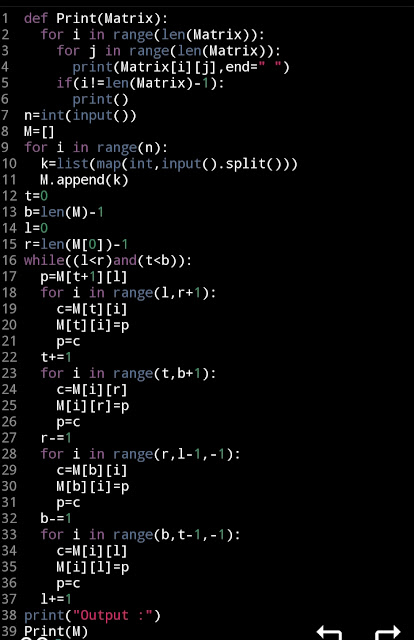
Output
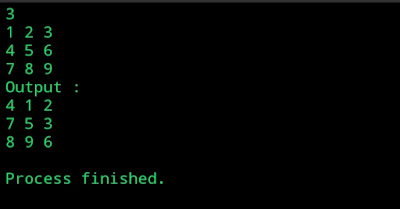
5. Missing number :
Given a list of n-1 numbers ranging from 1 to n , your task is to find the missing number. There are no duplicates.
Input format
The first line of the input contains n-1 numbers separated by space.
Output format
Print the missing number.
Sample input
1 2 4 6 3 7 8
Sample Output
5
Explanation
In the above list of numbers ranging from 1 to 8 the number 5 is missing. And hence 5 is the output.
Code
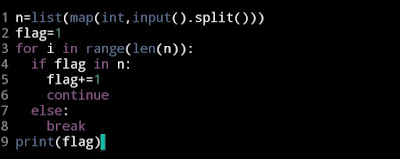
Output
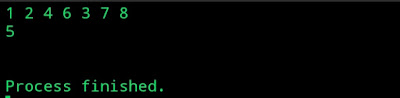
Related articles :
Comments
Post a Comment