Introduction to python(Installation of python,Python libraries,ASCII values,input and output statements)
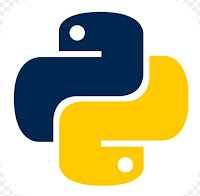
Installation of python:
Python is installed from c:\python27
Path variable has to be set in python file, inorder to run the program.
Python features:
- Python is very supportive for data analytics.
- It has general public licence (GPL).
- It is a scripting language.
- It is an object oriented programming language.
- It is an open source language.
Python libraries:
- NLP package.
- PIL(Python Image Library).
- Math
Functions:
1)print()
Use:It is used to print a statement or variable.
Example:
print ("hello world")
Output:
hello world
2)Data types:
- int
It includes all integers.eg.5,9,23 etc
- float
It includes all decimal values.eg. 2.7 ,4.0 etc
- str
It includes a group of alphabets.eg. hello ,won etc
3) type ()
Use:It is used to declare the data type.
Example:
X=3.0
Y=5
Z=X+Y
A=hello
print (type(X))
print (type(Y))
print (type(A))
Output:
Float
Int
String
4)type conversion:
The expression of one data type on other is called type conversion.
Example:
a=65
b=3.2
print (float(a))
print (int(b))
print (str(a))
Output:
65.0
3
A(ASCII code of A is 65)
ASCII :
A-65
B-66
C-67
D-68
E-69
F-70
G-71
H-72
I-73
J-74
K-75
L-76
M-77
N-78
O-79
P-80
Q-81
R-82
S-83
T-84
U-85
V-86
W-87
X-88
Y-89
Z-90
Zero-47
Space-30
5)input()
Use: It is used to provide input.
Example:
X=int(input())
print (X)
Output :
In this case we have to give the input and the input we give will be be printed as output.
6)raw_input()
It reads the input or command and returns a string.
Whereas input() reads the input and returns a python type like list ,tuple,int etc.
Comments
Post a Comment